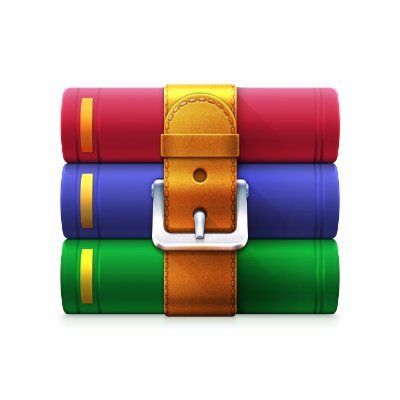
Variables are a fundamental concept in programming and are an essential part of the C++ language. Variables are used to store values in your program, and you can use them to perform calculations, store user input, and more.
To create a variable in C++, you first need to specify the type of the variable, such as an integer, float, or string. You then need to give the variable a name, which is a label you use to refer to the variable in your code. For example:
int age = 21;
float height = 5.7;
string name = "John Doe";
In this example, three variables are created: “age”, “height”, and “name”. The type of each variable is specified using the keyword “int”, “float”, and “string”, respectively. The value of each variable is assigned using the equal sign (=).
Once a variable has been created, you can use it in your program. For example:
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Height: " << height << endl;
In this example, the values of the variables “name”, “age”, and “height” are printed to the console.
Variables are also used to store values that can be changed during the execution of your program. For example:
int score = 0;
score = score + 1;
cout << "Score: " << score << endl;
In this example, the variable “score” is created with an initial value of 0. The value of “score” is then increased by 1 and the updated value is printed to the console.
Variables are a powerful tool in programming, and you’ll use them in nearly every program you write in C++. Understanding how to use variables is an essential step in becoming a skilled C++ programmer.
It’s also important to note that variables in C++ have scope. Scope refers to the area of the program where a variable is accessible. Variables declared inside a function are only accessible within that function, while variables declared outside of any function are accessible throughout the entire program.
Another important aspect of variables in C++ is type conversion. Type conversion is the process of converting a value from one data type to another. For example, you might want to convert an integer to a string, or a float to an int. There are two main types of type conversion in C++: implicit conversion and explicit conversion.
Implicit conversion occurs automatically, without any intervention from the programmer. For example, when you assign an int to a float, C++ will automatically convert the int to a float. This can be useful, but it can also lead to unexpected results if you’re not careful.
Explicit conversion, on the other hand, requires the programmer to specifically request the conversion. This is done using a cast, which is a special syntax in C++ that allows you to explicitly convert a value from one type to another. For example:
int x = 10;
float y = (float)x;
cout << "x: " << x << endl;
cout << "y: " << y << endl;
In this example, the integer value of x is explicitly cast to a float and stored in the variable y. The value of y is then printed to the console.
Finally, it’s worth mentioning that C++ has strict rules when it comes to naming variables. Variable names must start with a letter or underscore, and can only contain letters, numbers, and underscores. They can’t contain spaces, and they can’t be a reserved word in C++, such as “int” or “float”.
In conclusion, variables are an essential part of the C++ language and a fundamental concept in programming. Understanding how to create, use, and manipulate variables is an important step in becoming a skilled C++ programmer.