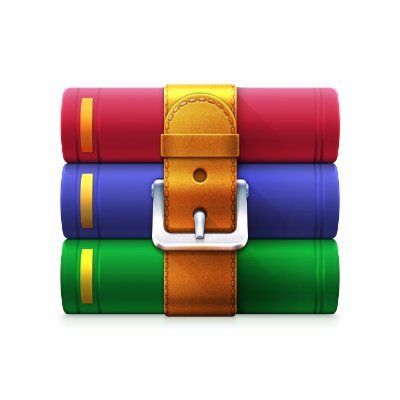
Python is one of the most popular programming languages in the world, and for good reason. It’s easy to learn, versatile, and used in a wide range of industries from finance to artificial intelligence. Whether you’re a complete beginner or looking to improve your skills, mastering Python is a valuable asset for any programmer.
At Ankitcodinghub.com, we understand the importance of learning Python and have created a comprehensive guide to help you master the language. Our guide is designed for beginners and covers all the essentials, including data types, variables, loops, and functions.
We start by introducing you to the basics of Python and its syntax, and then gradually build on that knowledge with more advanced topics such as object-oriented programming, modules, and file handling. We also include a range of practical examples and exercises to help you solidify your understanding of the concepts.
Our guide is not only helpful for those who are new to programming but also for those who are looking to improve their Python skills. We cover all the latest features of Python and provide you with the knowledge you need to write efficient and effective code.
If you’re looking to master Python, Ankitcodinghub.com is the perfect place to start. With our comprehensive guide, you’ll be coding like a pro in no time. So, don’t wait any longer, start your journey to mastering Python today!
Basic steps to learn python as beginner:
- Start with the basics: Learn the fundamental concepts of programming such as data types, variables, loops, and functions. You can find many resources online that offer free tutorials and exercises to help you get started.
- Practice, practice, practice: The more you practice coding, the more comfortable you will become with Python. Try to work on small projects or exercises to apply the concepts you learn.
- Learn the basics of object-oriented programming: Understanding the basics of OOP is essential for working with Python. Learn about classes, objects, inheritance, and polymorphism.
- Learn to use built-in modules and libraries: Python has a vast collection of built-in modules and libraries that can be used to perform various tasks. Learn to use them efficiently to write powerful code.
- Learn to work with files and data: Learn how to read and write files and how to work with different data formats such as CSV, JSON, and XML.
- Understand the role of debugging and testing: Learn how to debug and test your code to ensure it runs correctly and identify and fix errors.
- Join a community: Join a community of Python developers to learn from experienced programmers and to get help when you need it.
- Use resources like ankitcodinghub.com: Use resources like ankitcodinghub.com to access a wide range of tutorials, exercises, and projects to help you learn Python.
- Keep learning: Python is a constantly evolving language, and it’s important to keep learning new concepts and features to stay up-to-date.
- Practice and apply what you have learned: Practice and apply what you have learned to real-world projects and problems. This will give you a deeper understanding of the language and will help you become a better programmer.