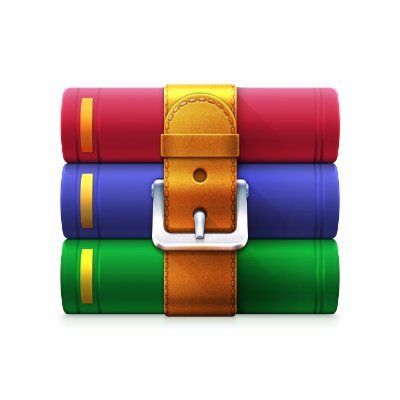
PHP Assignment Help | PHP Homework Help

PHP, or Hypertext Preprocessor, is a popular server-side scripting language designed for web development. Originally created in 1994 by Rasmus Lerdorf, PHP has evolved into a robust language that powers many of the world’s most popular websites and web applications.
PHP is a powerful language that offers a range of features, including the ability to create dynamic web pages, handle data input and output, and connect to databases. One of the biggest advantages of PHP is its ease of use, making it an ideal language for beginners and experienced developers alike.
PHP code is typically embedded in HTML code and executed on the server, generating dynamic content that can be displayed in a web browser. Some of the most common uses of PHP include web development frameworks, content management systems, e-commerce websites, and web-based applications.
At ankitcodinghub.com, we offer expert PHP homework help to students at all levels. Our team of experienced developers can help you with everything from basic PHP syntax to complex web applications and custom software development. Here are some examples of PHP coding assignments we have helped students with:
- E-commerce Website: We recently worked with a student who needed help developing an e-commerce website using PHP. We helped the student develop a custom shopping cart system, complete with product listings, shopping cart functionality, and checkout procedures. Our team also provided guidance on integrating payment gateways and other essential e-commerce features.
- Custom Web Application: Another student approached us with a unique project that required custom PHP development. The student needed to create a web-based application for tracking customer service requests, with the ability to assign requests to specific employees and track their progress. Our team helped the student design and develop a custom PHP application that met all of their requirements, including user authentication and access control.
- Database Connectivity: One of the most common uses of PHP is to connect to databases and retrieve data. We recently worked with a student who needed help retrieving data from a MySQL database using PHP. Our team helped the student write custom PHP code to connect to the database, retrieve the desired data, and display it on a web page.
In conclusion, PHP is a powerful and popular language for web development, and at ankitcodinghub.com, we have a team of experienced PHP developers who can help you with all of your PHP homework assignments. Whether you need help with basic syntax or complex web applications, our team has the expertise to help you succeed. Contact us today to learn more about our PHP homework help services.
here are some PHP coding examples and workouts:
- Example: Hello World!
The classic “Hello World!” program in PHP is simple and easy to write. Here is the code:
<?php
echo "Hello, World!";
?>
When you run this code in a web browser, you will see the output “Hello, World!”.
- Example: Variables and Data Types
PHP supports a variety of data types, including strings, integers, floating-point numbers, and booleans. Here is an example of how to define variables and use them in PHP:
<?php
$name = "John";
$age = 25;
$height = 6.2;
$is_student = true;
echo "Name: " . $name . "<br>";
echo "Age: " . $age . "<br>";
echo "Height: " . $height . "<br>";
echo "Is student? " . $is_student . "<br>";
?>
When you run this code in a web browser, you will see the output:
Name: John
Age: 25
Height: 6.2
Is student? 1
Note that the boolean variable $is_student
is printed as “1” because true is equivalent to the integer value 1 in PHP.
- Example: Arrays
PHP supports arrays, which can hold multiple values of the same or different data types. Here is an example of how to define and use arrays in PHP:
<?php
$fruits = array("apple", "banana", "cherry");
echo "First fruit: " . $fruits[0] . "<br>";
echo "Second fruit: " . $fruits[1] . "<br>";
echo "Third fruit: " . $fruits[2] . "<br>";
?>
When you run this code in a web browser, you will see the output:
First fruit: apple
Second fruit: banana
Third fruit: cherry
- Workout: Simple Calculator
In this workout, you will create a simple calculator that can perform addition, subtraction, multiplication, and division. Here is the code:
<!DOCTYPE html>
<html>
<head>
<title>Simple Calculator</title>
</head>
<body>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post">
<input type="number" name="num1" required>
<select name="operator" required>
<option value="+">+</option>
<option value="-">-</option>
<option value="*">*</option>
<option value="/">/</option>
</select>
<input type="number" name="num2" required>
<input type="submit" value="Calculate">
</form>
<?php
if (isset($_POST['num1']) && isset($_POST['num2']) && isset($_POST['operator'])) {
$num1 = $_POST['num1'];
$num2 = $_POST['num2'];
$operator = $_POST['operator'];
switch ($operator) {
case '+':
$result = $num1 + $num2;
break;
case '-':
$result = $num1 - $num2;
break;
case '*':
$result = $num1 * $num2;
break;
case '/':
$result = $num1 / $num2;
break;
default:
$result = "Invalid operator";
break;
}
echo "Result